👇 공부한 자료들의 실제 소스파일들을 기록해논 공간입니다 👇
GitHub - gayulz/StudyHistory: 나의 공부 기록 commit
나의 공부 기록 commit . Contribute to gayulz/StudyHistory development by creating an account on GitHub.
github.com
문제 1 . String 변수에 있는 숫자연산식에 연산을 처리하라
String a ="23 - 56 + 45 * 2 - 56";
수식을 계산하는 프로그램을 작성하세요.
수식 계산 규칙은 연산자 우선순위 없이 앞에서부터 차례대로 계산한다.
지금까지 공부한 내용으로 해결하시고, 형변환만 검색이 가능합니다.
package TASK;
public class task01 {
public static void main(String[] args) {
String a = "23-56+45*2-56";
String[] arrsub = new String[2];
String[] arr = new String[5];
char[] spChar = new char[4];
boolean flag = true;
int SubCnt = 0;
int arrT = 0;
int opCnt = 0;
// 숫자와 특수문자 분리 및 숫자 분해와 재조립 실행문
for (int i = 0; i < a.length(); i++) {
char v = a.charAt(i);
if (v == '-' || v == '*' || v == '+' || v == '%') {
spChar[opCnt] = v;
opCnt++;
flag = false;
} else {
arrsub[SubCnt] = Character.toString(v);
SubCnt++;
}
if (!flag) {
// 1자리 숫자가 들어올 경우 null값 없이 처리되게끔 진행
if (arrsub[1] == null) {
arr[arrT] = arrsub[0];
} else if (arrsub[1] == null && arrsub[2] == null) {
arr[arrT] = arrsub[0];
} else {
// null값이 없는 경우 join 연산으로 String 배열에 대입
arr[arrT] = String.join("", arrsub);
}
arrT++;
flag = true;
SubCnt = 0;
arrsub = new String[2];
}
// 마지막 숫자까지 배열처리
if (i == a.length() - 1) {
if (arrsub[1] == null) {
arr[arrT] = arrsub[0];
} else if (arrsub[1] == null && arrsub[2] == null) {
arr[arrT] = arrsub[0];
} else {
// null값이 없는 경우 join 연산으로 String 배열에 대입
arr[arrT] = String.join("", arrsub);
}
}
}
// 재사용 변수 초기화
opCnt = 0;
arrT = 0;
// 계산 진행
for (int i = 0; i < arr.length; i++) {
// 첫 숫자 대입
if (i == 0) {
arrT = Integer.parseInt(arr[i]);
}
if (i > 0) {
char op = spChar[opCnt];
if (op == '-') {
arrT = arrT - Integer.parseInt(arr[i]);
opCnt++;
} else if (op == '+') {
arrT = arrT + Integer.parseInt(arr[i]);
opCnt++;
} else if (op == '*') {
arrT = arrT * Integer.parseInt(arr[i]);
opCnt++;
} else if (op == '%') {
arrT = arrT % Integer.parseInt(arr[i]);
} else if (op == '/') {
arrT = arrT / Integer.parseInt(arr[i]);
}
}
// 연산 처리 결과 출력부
}
System.out.println("입력된 String 연산자 내용 : " + a);
System.out.println("연산 처리 결과 : " + arrT);
}
}
사실 해당 연산자 내용으로 하게되면 문제없이 처리되지만
숫자가 3자리로 바뀌었을때나 숫자가 더 많을 경우 문제가 생긴다(배열 크기에 대한 예외오류멘트)
수정해보려고 했으나 되지 않아 ㅜㅜ 명절이 끝나면 선생님에게 한번 여쭤봐야겠다.
문제 2. 가장 큰 수 구하기
int[] a = {34,2,35,8,31,45}
철수는 배열의 0번 인덱스부터 순회한다.
0번 인덱스에서는 0번 인덱스와 그다음 인덱스를 비교하여 큰 수를 뒤로 배치한다.
이러한 방식으로 배열의 뒤까지 반복하면 맨 뒤에 가장 큰 수가 배치 될 것이다.
위와 같은 방식으로 가장큰 수를 구하시오.
2중 for문을 사용한 정렬 정리
int[] a = {34,2,35,8,31,45};
for (int i = 0; i < a.length; i++) {
for (int j = 0; j < a.length; j++) {
int tmp = 0;
if (a[i] < a[j]) {
tmp = a[j];
a[j] = a[i];
a[i] = tmp;
}
}
}
큰 수를 비교하여 정렬을 처리한 뒤 마지막 인덱스를 출력하는 것으로 진행하려 하였다.
하지만 선생님에 한마디.
2중 for문을 쓰지 말라고 하셨다.
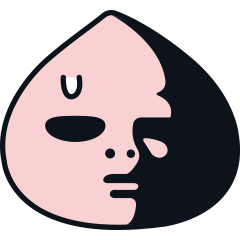
다시 풀기 시작했다.
// 선언부
int[] a = {70,2,35,45,31,1};
// 큰수 처리문
for (int i = 0; i < a.length; i++) {
int cnt = i+1;
if (a[i] > a[cnt]){
a[cnt] = a[i];
} else {
a[i] = a[cnt];
}
if(cnt == 5){
break;
}
}
// 출력문
System.out.print("가장 큰 수는 마지막 인덱스에 있다 : " + a[a.length-1]);
해당 코드로 진행하면
{ 70, 2, 35, 45, 31, 1 } 배열데이터가
{ 70, 70, 70, 70, 70, 70}으로 바뀌게 된다.
문제 3. 큰 수를 갱신하라
철수는 배열의 0번 인덱스에 가장 큰 값을 저장하면 될 것이라고 생각한다.
0번 인덱스가 가장 큰 값이라고 정의하고 1번부터 마지막까지 순회하면서
가장 큰 값이 나오면 가장 큰 값을 경신한다.
위와 같은 방식으로 가장 큰 수를 구하시오.
int[] arr = { 10, 2, 4, 7, 11, 4, 12};
for (int i = 0; i < arr.length; i++) {
int tmp = 0;
if ( arr[0] < arr[i] ){
tmp = arr[i];
arr[i] = arr[0];
arr[0] = tmp;
}
}
System.out.println("배열 중 가장 큰 값 : " + arr[0]);
System.out.println("배열의 인덱스별 값 ");
for (int i = 0; i < arr.length; i++) {
System.out.print("인덱스"+ i + " : " + arr[i] + "\n");
}
문제 4. 로또번호 추첨기
Random r = new Random();
변수이름은 r이며 랜덤 한 숫자를 추출하는 기능인 Random이라는 자료형을 선언함
int num = r.nextInt(45)+1;
변수 r을 참조하여 nextInt 메서드를 호출함. 매개변수는 랜덤한 숫자의 범위를 지정함
즉 0 - 44까지 중 랜덤한 숫자 하나를 선택하여 리턴함.
로또 번호는 1-45까지 이므로 리턴값이 +1로 보정함
리턴값을 보정하여 num변수의 값에 대입
위 코드를 이용하여
로또번호를 자동으로 생성하는 게임을 작성하시오.
중복허용 가능, 로또 번호 6개를 배열에 저장하고, 출력하시오.
// 선언부
Random r = new Random();
int[] lotto = new int[6];
// 로또번호 랜덤추출 입력 처리문
for (int i = 0; i < lotto.length; i++) {
lotto[i] = r.nextInt(45)+1;
}
System.out.println("로또번호 랜덤생성기");
System.out.println("--------------------");
// 로또번호 출력부
for (int i = 0; i < lotto.length; i++) {
System.out.println(i+1 + "번 번호 : " + lotto[i]);
}
문제 5. 로또 번호 추첨기에 중복값이 없도록 만들어보자
4번에서 중복이 되지 않게 하시오... 이때 for문은 한 개만 사용
// 선언부
Random r = new Random();
int[] lotto = new int[6];
// 로또번호 랜덤추출 입력 처리문
for (int i = 0; i < lotto.length; i++) {
lotto[i] = r.nextInt(45) + 1;
for (int j = 0; j < i; j++) {
if (lotto[i] == lotto[j]){
i--;
}
}
}
System.out.println("로또번호 랜덤생성기");
System.out.println("--------------------");
// 로또번호 출력부
for (int i = 0; i < lotto.length; i++) {
System.out.println(i + 1 + "번 번호 : " + lotto[i]);
}
문제 6. 가위 바위 보 게임
컴퓨터는 랜덤으로 번호를 먼저 뽑고, 키보드로 사용자가 번호를 입력하는 방식으로 가위 바위 보 게임을 작성하시오
// 선언문
Random r = new Random();
Scanner in = new Scanner(System.in);
int pc = r.nextInt(3);
// 게임시작 알림
System.out.println("가위바위보 게임 ");
System.out.println("주먹 : 0 , 가위 : 1, 보 : 2");
// User 입력값 확인 및 비교처리, 출력부
for (int i = 0; i < 1; i++) {
System.out.print("숫자를 입력 해 주세요 : ");
int user = in.nextInt();
if (user >= 3 || user < 0) {
System.out.println("주먹 : 0 , 가위 : 1, 보 : 2 만 입력 가능합니다");
i--;
}
if (pc == user) {
System.out.println("무승부 입니다. 다시하세요");
i--;
}
if (pc == 0) {
if (user == 1) {
System.out.println("PC는 주먹, User는 가위입니다");
System.out.println("PC 승리");
} else if ( user == 2 ){
System.out.println("PC는 주먹, User는 보입니다");
System.out.println("User 승리");
}
}
if (pc == 1) {
if (user == 0) {
System.out.println("PC는 가위, User는 주먹입니다");
System.out.println("User 승리");
} else if(user == 2) {
System.out.println("PC는 가위, User는 보입니다");
System.out.println("PC 승리");
}
}
if (pc == 2) {
if (user == 0) {
System.out.println("PC는 보, User는 주먹입니다");
System.out.println("PC 승리");
} else if(user == 1) {
System.out.println("PC는 보, User는 가위입니다");
System.out.println("User 승리");
}
}
}
문제 7. 로또번호 랜덤 추첨, 가장 중복 횟수가 큰 것을 구하라
컴퓨터는 랜덤으로 로또 번호를 10000번 뽑는다.
가장 많이 뽑힌 로또 번호가 오늘의 추천 로또 번호이다.
오늘의 추천 로또 번호 하나를 출력하시오. 만약 로또 번호가 같은 횟수로 나왔을 경우에는 큰 숫자가 우선한다.
package TASK;
import java.util.Random;
public class task07 {
public static void main(String[] args) {
Random r = new Random();
int[] lotto = new int[10000];
// 랜덤 추출 대입 배열
int[] CntNumber = new int[lotto.length];
// 카운팅 된 배열
int numPoint = 0;
// 비교하는 대상 번호의 위치 포인터 번호 _ 1번 더 재사용함
int cnt = 0;
// 카운트 갯수 사용 변수 _ 1번더 재사용함
int maxNumber = 0;
// 결과 출력 변수
// 랜덤 수 대입문
for (int i = 0; i < lotto.length; i++) {
lotto[i] = r.nextInt(45) + 1;
}
// 0~10000번까지의 중복카운트 및 번호 확인
for (int i = 0; i < lotto.length; i++) {
int tmp = lotto[numPoint];
// i번 인덱스부터 ~ 마지막까지 지속 비교
if (tmp == lotto[i]) {
cnt++;
}
if ( i == lotto.length-1 ){
CntNumber[numPoint] = cnt;
numPoint++;
i=0;
cnt = 0;
}
// 배열에 넣은 갯수와 lotto배열 크기-1과 같아질 경우 종료
if( numPoint == lotto.length-1){
CntNumber[numPoint] = cnt;
numPoint=0;
cnt=0;
break;
}
}
// 배열별 카운트가 가장 높은 숫자 추출
for (int i = 0; i < CntNumber.length; i++) {
if (cnt < CntNumber[i]){
cnt = CntNumber[i];
// 큰 값을 cnt에 입력해 놓는다
numPoint = i;
// 큰 카운트 숫자의 인덱스 번호를 넣어준다
}
if ( cnt == CntNumber[i]){
// 큰 번호와 i인덱스의 데이터값이 같을 경우
if (lotto[numPoint] > lotto[i] ){
// cnt 데이터의 인덱스번호를 사용하여 lotto숫자를불러오고,
// i를 사용하여 lotto숫자를 불러와 비교한다
maxNumber = lotto[numPoint];
} else if (lotto[numPoint] < lotto[i] ){
maxNumber = lotto[i];
} else if (lotto[numPoint] == lotto[i] ){
maxNumber = lotto[i];
}
}
}
System.out.println(" 행운의 로또번호 추첨기, 번호 1개만 제공합니다 . ");
// cnt = 가장 높은 카운트 횟수 ,
// numPoint = 해당 인덱스 번호
System.out.println(" 가장 높은 중복 횟수 : " + cnt );
System.out.println(" 오늘의 번호 : " + maxNumber);
}
}
과제 후기
노베이스 친구들 보다는 그래도 컴퓨터공학과 사이버대로 졸업예정인 내가 문제 푸는 게 너무 느린 것 같다
나는 이렇게 2일 동안 끙끙대며 풀었던 문제를, 어떤 친구는 그날 다 푸는 모습을 보고
아직 나는 많이 부족한가 보다 싶었다.
자극받아서 더 열심히 공부해야겠다 파이팅!!
'👩🏻💻 𝐋𝐚𝐧𝐠𝐮𝐚𝐠𝐞 > ⠀⠀⠀⠀ Jᴀᴠᴀ' 카테고리의 다른 글
2023/10/05🐰 중첩FOR문 (0) | 2023.10.10 |
---|---|
2023/10/04✨ break & continue (1) | 2023.10.04 |
2023/09/26🤔 반복문과 배열 문제 풀기 (0) | 2023.09.29 |
2023/09/25🌙 배열 및 for문 활용 (0) | 2023.09.26 |
2023/09/22🌹 단일 for 문과 if 문 실습 (0) | 2023.09.23 |